Strategy Design Pattern in Java
This article explains strategy design pattern in Java with class diagrams and example code.
Introduction
Strategy Design Pattern is a behavioral design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. I.e. the strategy design pattern deals with how the classes interact with each other.
What is Strategy Design Pattern
Strategy Pattern is used when there is a family of interchangeable algorithms of which one algorithm is to be used depending on the program execution context.
Strategy Pattern achieves this by clearly defining the family of algorithms, encapsulating each one and finally making them interchangeable. Each of the algorithms in the family can vary independently from the clients that use it.
Interchangeability is achieved by making a parent interface/base strategy class and keeping individual algorithms as children of this base class. The client class holds a reference to the base strategy and at runtime receives the correct algorithm child instance depending on the execution context.
Lets have a look at the class diagram of the strategy pattern -
Strategy Design Pattern Class Diagram
Explanation of Class Diagram
Explanation of Code & Class Diagram
The Java Example's Class Diagram is for a family of algorithms for file parsers where -
Summary
This concludes the article on strategy design pattern where we looked at what is the pattern, pattern class diagram and its explanation and finally an example of the pattern in java with class diagram and sample code.
Lets have a look at the class diagram of the strategy pattern -
Strategy Design Pattern Class Diagram
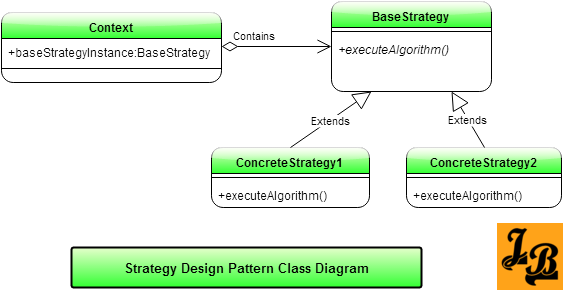
BaseStrategy
class is an abstract class which represents the algorithm.executeAlgorithm()
is the abstract method inBaseStrategy
which is responsible for executing the algorithm, but is puposefully kept abstract so that each of the child strategies/algorithms can implement this method with their own logic.ConcreteStrategy1
&ConcreteStrategy2
are two subclasses ofBaseStrategy
. These 2 are infact two different variants of the algorithm represented by theBaseStrategy
.ConcreteStrategy1
&ConcreteStrategy2
overrideexecuteAlgorithm()
method fromBaseStrategy
to provide the implementation of the algorithm variant as per their own needs.- Lastly, the
Context
class invokes this algorithm. Based on the need of the execution context specific variant of theBaseStrategy
- either ofConcreteStrategy1
orConcreteStrategy2
is assigned to thebaseStrategyInstance
variable ofContext
. Context
always invokesbaseStrategyInstance
variable'sexecuteAlgorithm()
method, but the actual algorithm which is executed is based on which instance ofConcreteStrategy
is held bybaseStrategyInstance
.
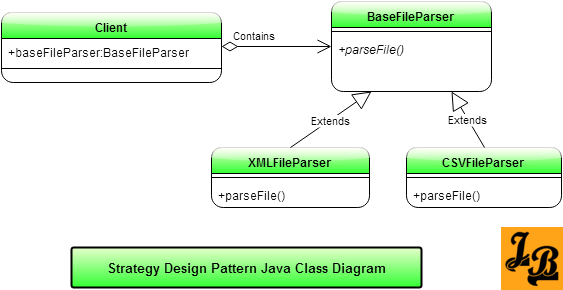
Code for the classes shown in Java Example’s Class Diagram
//BaseFileParser.java
public abstract class BaseFileParser{
public abstract void parseFile();
}
//XMLFileParser.java
public class XMLFileParser extends BaseFileParser{
public void parseFile(){
//Logic for parsing an XML file goes here
}
}
//CSVFileParser.java
public class CSVFileParser extends BaseFileParser{
public void parseFile(){
//Logic for parsing a CSV file goes here
}
}
//Client.java
public class Client{
private BaseFileParser baseFileParser;
public Client(BaseFileParser baseFileParser){
this.baseFileParser=baseFileParser;
}
public void parseFile(){
baseFileParser.parseFile();
}
public static void main(String args[]){
//Lets say the client needs to parse an XML file
//The file type(XML/CSV) can also be taken as
//input from command line args[]
Client client=new Client(new XMLFileParser());
client.parseFile();
}
}
BaseFileParser
class is an abstract base class which represents family ofFileParser
instances in the design. It has a single abstract methodparseFile()
which needs to be invoked to parse a file. This method will be overridden by individual parsers next.XMLFileParser
&CSVFileParser
both implementBaseFileParser
. Both also overrideparseFile()
method as per their own logic for processing information in XML and CSV formats.Client
class needs to parse files and hence is a consumer ofFileParser
instances.Client
class holds an instance ofBaseFileParser
which is initialized with the correct subtype ofXMLFileParser
orCSVFileParser
. This happens at the time of instantiation of theClient
using a parameterized constructor which assigns the correct file parser to thebaseFileParser
variable.- When
Client
class'sparseFile()
method is invoked, it in turn invokesparseFile()
method of the child ofBaseFileParser
(XMLFileParser
orCSVFileParser
) which is contained inbaseFileParser
variable at that moment. - Thus, appropriate CSV or XML file parsing happens based on the context.
- As you might have noticed in the
main()
method that the information of which child parser(CSV or XML) is to be assigned to theClient
’sbaseFileParser
variable will be passed at runtime. This information can also be passed through the command line argumentsargs[]
- So,
Client
is able to parse the incoming file by selecting at runtime one of the strategies of CSV or XML based. - If another file format, say PDF, needs to be implemented in future then all we need is to create a new subclass of
BaseFileParser
calledPDFFileParser
and implement PDF file handling logic in itsparseFile()
method.
Gang of Four (GOF) Design Patterns on JavaBrahman
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern