Adapter Design Pattern in Java
This article explains adapter design pattern in java with class diagrams and example code.
Introduction Adapter class makes classes with incompatible interfaces work together. Adapter Design Pattern is a structural design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. I.e. the adapter pattern deals with how the classes are composed to form larger structures. What is Adapter Design Pattern When the target class has an interface which is different from what the client class expects, then adapter pattern solves this problem by converting the interface of the target into the client expected one. As a result classes which otherwise would not have been able to communicate with each other can now communicate with ease. Variants of Adapter Design Pattern Adapter design pattern has 2 variants -
Note - In the class diagram above I have focussed on showing the primary participating classes & their methods. I have not included the method parameters and constructors in the same.
Code for the classes drawn in Java Class Diagram
Explanation of the code & class diagram
Java Class Diagram is for file handling classes which take care of committing/emailing of files -
Summary
This concludes the article on adapter design pattern where we saw what is adapter pattern. Then we learned about its two variants - Class and Object Adapters and saw class diagram of each. Then we saw a Java Implementation of a FileEmailer Adapter through code and then its explanation.
Introduction Adapter class makes classes with incompatible interfaces work together. Adapter Design Pattern is a structural design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. I.e. the adapter pattern deals with how the classes are composed to form larger structures. What is Adapter Design Pattern When the target class has an interface which is different from what the client class expects, then adapter pattern solves this problem by converting the interface of the target into the client expected one. As a result classes which otherwise would not have been able to communicate with each other can now communicate with ease. Variants of Adapter Design Pattern Adapter design pattern has 2 variants -
- Class Adapters: Class Adapter works by subclassing both the Target and the Adaptee. Adapter overrides the Target interface methods such that every client call made on the overridden target interface is redirected to the Adaptee's inherited method which does the actual operation. Class Adapter's class diagram is as shown below -
Adapter
inherits both fromTarget
andAdaptee
.- The client invokes
requiredMethod()
on an instance ofAdapter
which is also an instance ofTarget
by virtue of being its subclass. Adapter
overrides therequiredMethod()
ofTarget
and in its implementation internally callsAdaptee
’stargetMethod()
.- Thus,
Client
is able to usetargetMethod()
functionality fromAdaptee
through theAdapter
without change of the interface which still remainsTarget
for the client.
- Object Adapters: Object Adapter works by composing the Adaptee and extending the Target. Adapter overrides the Target interface methods such that every client call made to the overridden target interface's method is redirected to the composed Adaptee object's method which does that operation. Let's have a look at the class diagram for Object Adapters -
Adapter
extendsTarget
.Adapter
also keeps an instance ofAdaptee
as a class variable.- When
Client
calls - - The
Client
invokesrequiredMethod()
on an instance ofAdapter
which is also an instance ofTarget
by virtue of being its subclass. Adapter
overrides therequiredMethod()
ofTarget
and in its implementation internally calls its class variable adaptee'stargetMethod()
.- Thus,
Client
is able to usetargetMethod()
functionality fromAdaptee
through theAdapter
without change of the interface which still remainsTarget
for the client.
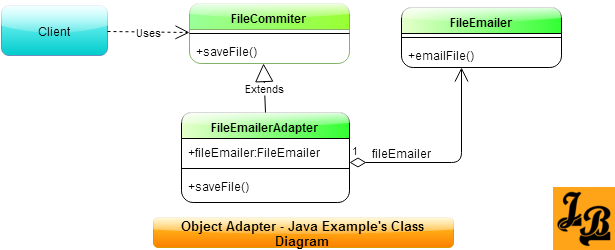
Code for the classes drawn in Java Class Diagram
Code for classes shown in Java Class Diagram
//FileCommiter.java
import java.io.File;
public class FileCommiter {
String diskLocation;
public FileCommiter(){
//default constructor
}
public FileCommiter(String diskLocation){
this.diskLocation=diskLocation;
}
public void saveFile(File file){
//Logic for saving the file at the diskLocation goes here
}
}
//FileEmailer.java
import java.io.File;
public class FileEmailer {
public String emailAddress;
public FileEmailer(String emailAddress){
this.emailAddress=emailAddress;
}
public void emailFile(File file){
//Logic for emailing the file goes here
}
}
//FileEmailerAdapter.java
import java.io.File;
public class FileEmailerAdapter extends FileCommiter{
public FileEmailer fileEmailer;
public FileEmailerAdapter(FileEmailer fileEmailer){
this.fileEmailer=fileEmailer;
}
public void saveFile (File file){
this.fileEmailer.emailFile(file);
}
}
//Client.java
import java.io.File;
public class Client{
public static void main(String args[]){
File file=new File(args[0]);
FileCommiter fileCommiter=new FileCommiter("C:\\");
fileCommiter.saveFile(file);//This will save the file on the disk location C: drive
//Now we want to email with the same Target class FileCommiter
fileCommiter=new FileEmailerAdapter(new FileEmailer("abcd@javabrahman.com"));
fileCommiter.saveFile(file);//This will email the file to abcd@javabrahman.com
}
}
FileCommiter
class has the responsibility of committing files to the disk location. The disk location is set via a constructor parameter.FileEmailer
class has the responsibility of emailing files to the email address. The email address is set via a constructor parameterFileEmailerAdapter
extendsFileCommiter
.FileEmailerAdapter
has a class variable of typeFileEmailer
calledfileEmailer
. ThisfileEmailer
class variable is set via a constructor parameter.- Client first creates a new
java.io.File
instance based on command line argument passed to it. - Client then creates a
FileCommiter
instance with a disk location value of C drive and saves the file instance it created using thesaveFile()
method. - Next the Client creates a
FileEmailerAdapter
instance using aFileEmailer
with an email address. - Client then invokes the
saveFileMethod()
onfileEmailAdapter
. This time instead of commiting the file on the disk locationFileEmailerAdapter
internally routes the request toemailFile()
method of class variableadaptee
. - Thus, with the same interface of
FileCommiter
, Client is now able to email a file as well.This is adapter pattern.
Gang of Four (GOF) Design Patterns on JavaBrahman
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern