Builder Design Pattern in Java
This tutorial explains Builder design pattern in java with UML class diagram. It then takes an example scenario in java and explains it with class diagram and code.
Introduction Builder Design Pattern is a creational design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. Being a creational design pattern, the Builder pattern deals with the design of how the object creation is managed. What is Builder Design Pattern In a Builder Design Pattern implementation the construction of a complex object is separated from its representation. This enables us to create multiple representations using the same construction process. In Builder Pattern the class which invokes the the multiple steps to create sub-parts of the final product is loosely coupled to the actual implementation of those steps using an abstract Builder interface in between. Scenarios in which Builder Design Pattern can be used Given below are the two conditions which together must be applicable to justify the use of Builder pattern while creating a product -
Class Diagram for Builder Design Pattern
Explanation of Builder Design Pattern's Class Diagram
OUTPUT on running Client.java is
Explanation of Java Example's Class Diagram & Code
The Java class diagram above depicts Builder Design pattern implemented for a Document Creation Engine. Lets quickly go through whats there in Java's example's class diagram & corresponding code -
Summary
In the above tutorial we understood what is Builder design pattern and the main scenarios in which it is applicable. We then looked at the UML class diagram for Builder Design Pattern & its explanation, a Java Use Case implementing Builder pattern with its class diagram and code for the classes shown in the class diagram, followed by explanation of both the class diagram & code. This concludes the tutorial on Builder design pattern.
Introduction Builder Design Pattern is a creational design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. Being a creational design pattern, the Builder pattern deals with the design of how the object creation is managed. What is Builder Design Pattern In a Builder Design Pattern implementation the construction of a complex object is separated from its representation. This enables us to create multiple representations using the same construction process. In Builder Pattern the class which invokes the the multiple steps to create sub-parts of the final product is loosely coupled to the actual implementation of those steps using an abstract Builder interface in between. Scenarios in which Builder Design Pattern can be used Given below are the two conditions which together must be applicable to justify the use of Builder pattern while creating a product -
- Algorithm for creating the product needs to be independent from the sub-parts' creation & assembly: Builder pattern is useful when the algorithm/steps for creation of a final complex product needs to vary independent of the sub-components for that product which are created and then assembled to get the desired product.
- Different representations of the product are possible: The product being created has variants which are different representations of the same basic product.
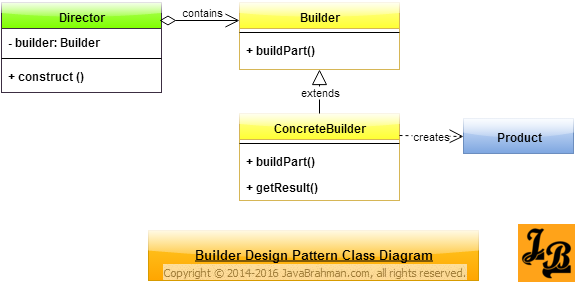
Builder
is the base class which all theConcreteBuilder
instances extend.Director
holds a reference to aBuilder
.Director
directs,i.e. is responsible for, creation of the product using the interface provided to it byBuilder
.- Each
ConcreteBuilder
is responsible for a variant of the product. WhilebuildPart()
method triggers the creation of sub-part, thegetResult()
method is provided to fetch the final assembled product. Product
object represents the complex final product which is being constructed.
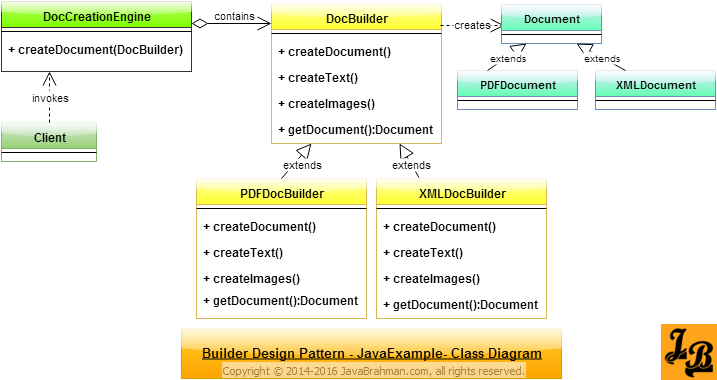
Code for the classes shown in Java Example’s Class Diagram
//Interface - Document.java
public interface Document{
}
//Class PDFDocument.java
public class PDFDocument implements Document{
//attributes for holding the PDFDocument
}
//Class XMLDocument.java
public class XMLDocument implements Document{
//attributes for holding the XMLDocument
}
//Abstract class - DocBuilder.java
public abstract class DocBuilder{
public abstract void createDocument();
public abstract void createText();
public abstract void createImages();
public abstract Document getDocument();
}
//PDFDocBuilder.java
public class PDFDocBuilder extends DocBuilder{
private PDFDocument pdfDoc;
public void createDocument(){
this.pdfDoc=new PDFDocument();
}
public void createText(){
System.out.println("Creating text for PDF Document.");
}
public void createImages(){
System.out.println("Creating images for PDF Document.");
}
public Document getDocument(){
System.out.println("Fetching PDF Document.");
return this.pdfDoc;
}
}
//XMLDocBuilder.java
public class XMLDocBuilder extends DocBuilder{
private XMLDocument xmlDoc;
public void createDocument(){
this.xmlDoc=new XMLDocument();
}
public void createText(){
System.out.println("Creating text for XML Document.");
}
public void createImages(){
System.out.println("Creating images for XML Document.");
}
public Document getDocument(){
System.out.println("Fetching PDF Document.");
return this.xmlDoc;
}
}
//DocCreationEngine.java
public class DocCreationEngine{
public void generateDocument(DocBuilder builder){
builder.createDocument();
builder.createText();
builder.createImages();
}
}
//Client.java
public class Client{
public static void main(String args[]){
DocCreationEngine engine=new DocCreationEngine();
//Creating PDF Document
PDFDocBuilder pdfDocBuilder=new PDFDocBuilder();
engine.generateDocument(pdfDocBuilder);
PDFDocument pdfDocument=(PDFDocument)pdfDocBuilder.getDocument();
//Creating XML Document
XMLDocBuilder xmlDocBuilder=new XMLDocBuilder();
engine.generateDocument(xmlDocBuilder);
XMLDocument xmlDocument=(XMLDocument)xmlDocBuilder.getDocument();
}
}
Creating text for PDF Document. Creating images for PDF Document. Fetching PDF Document. Creating text for XML Document. Creating images for XML Document. Fetching XML Document.
Document
is the base class for all documents.PDFDocument
&XMLDocument
are two types of Documents.DocBuilder
is the base class for Builders. It defines the methods whichDocCreationEngine
invokes to create a document.PDFDocBuilder
andXMLDocBuilder
are concrete builders for PDF and XML Documents respectively.DocCreation
engine is the Director, i.e. it invokes the methods onDocBuilder
instance passed to and creates the required document.Client
invokes theDocCreationEngine
with the specificDocBuilder
concrete class depending on the type of document it wants to generate.- Once the document has been created,
Client
callsgetDocument()
method on the concrete builder instance to get the PDF or XML document. - Output shows the sequence of creation of PDF and XML Documents.
Gang of Four (GOF) Design Patterns on JavaBrahman
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern