Prototype Design Pattern in Java
This tutorial explains Gang of Four's Prototype design pattern in java with UML class diagram. It explains the scenarios in which Prototype design pattern can be applied, and then implements an example use case in Java which shows how the Prototype pattern can be applied to a real world example. It shows the design of the use case using a class diagram, provides the Java code for the use case and lastly explains the working of the code.
Introduction Prototype Design Pattern is a creational design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. Being a creational design pattern, the Prototype pattern deals with the design of how the object creation is managed. What is Prototype Design Pattern In a Prototype Design Pattern implementation the type of object to be created is specified using a prototypical instance. New objects are created by copying(or cloning) this prototype. In simpler terms, in Prototype Pattern we keep an object of the type we want to create and any new object is created by simply cloning this existing object. Scenarios in which Prototype Design Pattern can be used
Class Diagram for Prototype Design Pattern
Explanation of Prototype Design Pattern's Class Diagram
OUTPUT on running UMLTool.java
Explanation of Java Example’s Class Diagram & Code
The Java class diagram above depicts Prototype Design pattern implemented for few elements of Class Diagram of a UMLTool viz. Classes, attributes and association. Lets quickly go through whats there in Java's example's class diagram & corresponding code -
Introduction Prototype Design Pattern is a creational design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. Being a creational design pattern, the Prototype pattern deals with the design of how the object creation is managed. What is Prototype Design Pattern In a Prototype Design Pattern implementation the type of object to be created is specified using a prototypical instance. New objects are created by copying(or cloning) this prototype. In simpler terms, in Prototype Pattern we keep an object of the type we want to create and any new object is created by simply cloning this existing object. Scenarios in which Prototype Design Pattern can be used
- Exact class to be instantiated is specified dynamically: When the class of which instance is to be created is specified at runtime, then Prototype pattern can be used.
- Object creation using 'new' keyword is costlier: When the inherent cost of object creation using 'new' keyword is more than simply cloning an existing standard object.
- When Abstract Factory-like class hierarchy is not required: To avoid maintaining an abstract factory like class hierarchy of multiple factories.
- Object States can be pre-defined: If the objects to be created can only have one of a finite number of states, then keeping prototypes of these objects and cloning them when needed is more convenient
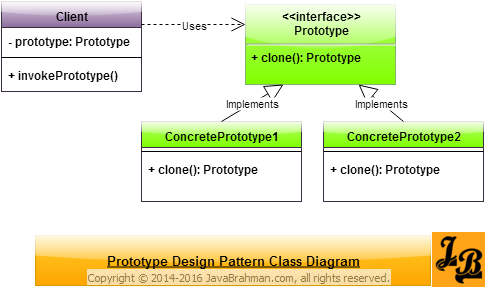
Prototype
is the base interface with a single methodclone()
.- Every concrete class which can be cloned must extend
Prototype
and override theclone()
method. - Every child of
Prototype
implements its own cloning logic in the overridenclone()
method. Client
invokes the clone method of the concrete child ofPrototype
and gets a cloned object back.
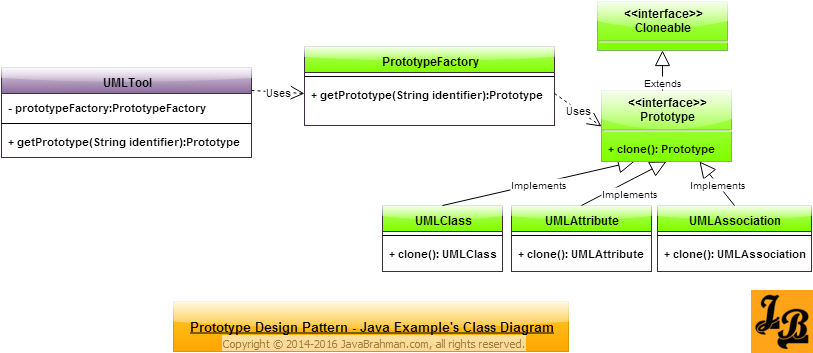
Code for the classes shown in Java Example's Class Diagram
//Prototype.java
public interface Prototype extends Cloneable{
public Prototype clone() throws CloneNotSupportedException;
}
//UMLClass.java
public class UMLClass implements Prototype{
private String className="Default Prototype Class Name";
public void setClassName(String className){
this.className=className;
}
public String getClassName(){
return this.className;
}
@Override
public UMLClass clone() throws CloneNotSupportedException{
System.out.println("Creating clone of UMLClass");
return (UMLClass) super.clone();
}
}
//UMLAttribute.java
public class UMLAttribute implements Prototype{
private String attributeName="Default Prototype Attribute Name";
public void setAttributeName(String attributeName){
this.attributeName=attributeName;
}
public String getAttributeName(){
return this.attributeName;
}
@Override
public UMLAttribute clone() throws CloneNotSupportedException {
System.out.println("Creating clone of UMLAttribute");
return (UMLAttribute) super.clone();
}
}
//UMLAssociation.java
public class UMLAssociation implements Prototype{
private String associationName="Default Prototype Association Name";
public void setAssociationName(String associationName){
this.associationName=associationName;
}
public String getAssociationName(){
return this.associationName;
}
@Override
public UMLAssociation clone() throws CloneNotSupportedException {
System.out.println("Creating clone of UMLAssociation");
return (UMLAssociation) super.clone();
}
}
//PrototypeFactory.java
import java.util.HashMap;
public class PrototypeFactory{
private static HashMap<String,Prototype> cloneMap=new HashMap<String,Prototype>();
static{
cloneMap.put("Class", new UMLClass());
cloneMap.put("Attribute", new UMLAttribute());
cloneMap.put("Association", new UMLAssociation());
}
public Prototype getPrototype(String identifier) throws CloneNotSupportedException{
switch(identifier){
case "Class": return cloneMap.get("Class").clone();
case "Attribute": return cloneMap.get("Attribute").clone();
case "Association": return cloneMap.get("Association").clone();
}
return null;
}
}
//UMLTool.java
public class UMLTool{
public static void main(String args[]){
try{
//Creating a Prototype of UMLClass
UMLClass umlClass=(UMLClass)new PrototypeFactory(). getPrototype("Class");
System.out.println("Name in umlClass instance:"+ umlClass.getClassName());
//Creating a Prototype of UMLAttribute
UMLAttribute umlAttrb=(UMLAttribute)new PrototypeFactory(). getPrototype("Attribute");
System.out.println("Name in umlAttrb instance:"+ umlAttrb.getAttributeName());
//Creating a Prototype of UMLAssociation
UMLAssociation umlAssoc=(UMLAssociation)new PrototypeFactory(). getPrototype("Association");
System.out.println("Name in umlAssoc instance:"+ umlAssoc.getAssociationName());
}catch(CloneNotSupportedException cloningException){
System.out.println("error in cloning:");
cloningException.printStackTrace();
}
}
}
Creating clone of UMLClass Name in umlClass instance:Default Prototype Class Name Creating clone of UMLAttribute Name in umlAttrb instance:Default Prototype Attribute Name Creating clone of UMLAssociation Name in umlAssoc instance:Default Prototype Association Name
UMLTool
is the client class.Prototype
is the base interface which all the three classes we need to clone implement.UMLClass
,UMLAttribute
andUMLAssociation
are three classes we will clone.PrototypeFactory
helps in cloning as there are multiple children ofPrototype
which are to be cloned. It returns an instance of the appropriate cloned object based on theString
identifier passed to it.PrototypeFactory
stores a staticHashMap
which contains one already instantiated object of three concrete classes which are cloneable.- UMLTool's
main()
method clonesUMLClass, UMLAttribute
&UMLAssociation
once each and then prints the value of their name variable. As you can see in the output that first a cloned instance for each of them is created and then the name attribute's value is correctly printed, as was stored in their instance stored in static map inPrototypeFactory
.
Gang of Four (GOF) Design Patterns on JavaBrahman
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern