Observer Design Pattern in Java
This article explains Observer design pattern in java with UML class diagram. It then takes an example scenario in java and explains it with class diagram and code.
Introduction
Observer Design Pattern is a behavioral design pattern among the Gang Of Four(GOF) Design PatternsArticle on GOF Patterns & their types. Being a behavioral design pattern, the Observer pattern deals with how objects of the designed system interact with each other.
What is Observer Design Pattern
Observer Design Pattern is used when there are multiple subscribers observing updates from a publisher. The publisher, known as Subject, publishes its change of state to its subscribers. Subscribers, known as Observers, receive these changes and update themselves accordingly.
Scenarios in which Observer Design Pattern can be used
Class Diagram for Observer Design Pattern
Explanation of Observer Design Pattern's Class Diagram
The following class diagram shows the relationship between
Lets now look at an example implementation of observer design pattern in Java using
Observer Design Pattern Example Implementation in Java-Class Diagram
Code for the classes shown in Java Example's Class Diagram
OUTPUT on running Client.java
Explanation of Java Example's Class Diagram & Code
The Java class diagram above depicts Observer Design pattern implemented for a simple Publish-Subscribe implementation. Let us now go through whats there in Java's example's class diagram & corresponding code -
Summary
In the above tutorial we understood what is Observer design pattern and the main scenarios in which this pattern is applicable. We then looked at the UML class diagram for Observer Design Pattern & its explanation, a Java Use Case implementing Observer pattern with its class diagram and code for the classes shown in the class diagram, followed by explanation of both the class diagram & code. This concludes the tutorial on Observer design pattern.
- Change of an object requires changes to multiple others: When there is an observed object(a.k.a the Subject), then there should be a mechanism to notify its changes to multiple of its observer components which have evinced interest in listening to these changes without the exact quantity of observers being known at design time.
- Notification capability needed without tight rules regarding how this notification is used: When the different Observers of notifications from a Subject have there own presentation and\or business logic implementation, but still need to have the capability of getting the latest updates from Subject. I.e. Observers are loosely coupled with the Subject
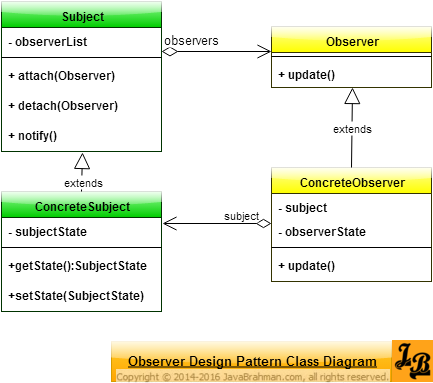
Subject
is the one being observed. It hasattach()
&detach()
methods to add or remove observers respectively. It usesnotify()
method to publish its changes to observers\subscribers.Observer
objects register\attach themselves to theSubject
they want to listen to for updates.ConcreteObserver
instances are actualObserver
objects which treat the notifications they receive from theSubject
in their own individual ways.ConcreteSubject
is the actualSubject
object whose state change is notified to multiple observers.
java.util.Observable
(represents Subject) and the interface java.util.Observer
(represents an Observer). Concrete Observers in Java need to implement the Observer
interface, whereas concrete Subject needs to extend Observable
to provide its own notification logic. The following class diagram shows the relationship between
Observer
and Observable
as defined in the Java SDK follows - 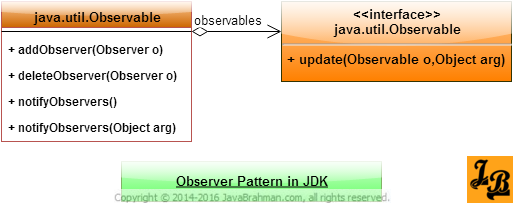
Observer
and Observable
.Observer Design Pattern Example Implementation in Java-Class Diagram
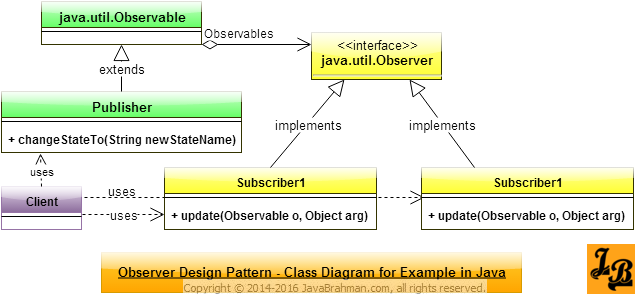
Code for the classes shown in Java Example's Class Diagram
Java code for implementing the example shown in class diagram above
//Publisher.java
import java.util.Observable;
public class Publisher extends Observable{
public void changeStateTo(String newStateName){
this.setChanged();
this.notifyObservers(newStateName);
}
}
//Subscriber1.java
package com.javabrahman.java8.designpatterns.observer;
import java.util.Observable;
import java.util.Observer;
public class Subscriber1 implements Observer{
String currentPublisherState;
@Override
public void update(Observable o, Object arg) {
System.out.println("New state received by Subscriber 1:"+arg.toString());
this.currentPublisherState=(String)arg;
}
}
//Subscriber2.java
import java.util.Observable;
import java.util.Observer;
public class Subscriber2 implements Observer{
String currentPublisherState;
@Override
public void update(Observable o, Object arg) {
System.out.println("New state received by Subscriber 2:"+arg.toString());
this.currentPublisherState=(String)arg;
}
}
//Client.java
public class Client {
public static void main(String args[]){
Publisher publisher=new Publisher();
publisher.addObserver(new Subscriber1());
publisher.addObserver(new Subscriber2());
publisher.changeStateTo("assigned");
}
}
New state received by Subscriber 1: assigned New state received by Subscriber 2: assigned
Publisher
is the Subject. It extendsjava.util.Observable
.Subscriber1
&Subscriber2
are the Observers. They implementjava.util.Observer
.Client
first initiatesPublisher
. It then adds one instance each ofSubscriber1
&Subscriber2
to Publisher's list of Observers.Client
then invokes methodchangeStateTo()
with new state value as "assigned". InternallyPublisher
then initiatesnotifyObservers()
with this new state value. Before notifying Publisher callssetStateChanged()
which is a requirement of the java's observer pattern implementation.update()
methods of Subscriber1 and Subscriber 2 are called internally by thenotifyObservers()
method and the new state value received by both in the parameterarg
is printed.
Gang of Four (GOF) Design Patterns on JavaBrahman
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern