Facade Design Pattern in Java
This tutorial explains Facade Design Pattern in Java with class diagrams and example code.
Introduction Facade Design Pattern is a structural design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. Being a structural design pattern, the facade pattern basically deals with how the classes are composed together to get the desired outcome from a system. What is Facade Design Pattern The basic design problem which the facade pattern solves is to simplify access to a set of interfaces in a subsystem. Facade solves this problem by providing a higher-level interface one level above all the sub-level interfaces. This higher-level interface then deals with the details and intricacies of the subsystem interface and at the same time provides a simplified interfaces to the clients of the subsystem.
Facade pattern improves decoupling between the clients and subsystems as the higher-level interface can absorb any changes which might happen in the lower subsystems interfaces and provide a consistent interface to the clients. This also has a direct benefit of system portability as the clients only design and code for the higher-level interface defined by the Facade. Porting of the subsystems then becomes easy as all the complexity of porting is hidden from the clients by the Facade layer.
Layered subsystems can have a facade for each layer. Thus communication between layers of the subsystem is much simplified as it happens through the subsystem facades rather than directly deal with the complexities of the subsystem's individual classes.
Class Diagram for Facade Design Pattern
Explanation of Class Diagram
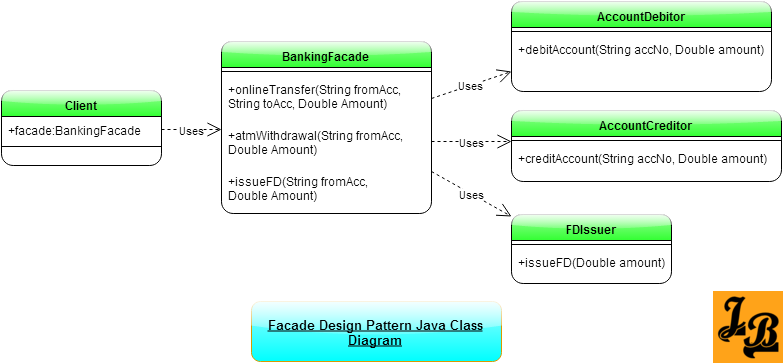
Explanation of Code & Class Diagram
The Java class diagram above depicts facade pattern implemented for a banking system where -
Introduction Facade Design Pattern is a structural design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. Being a structural design pattern, the facade pattern basically deals with how the classes are composed together to get the desired outcome from a system. What is Facade Design Pattern The basic design problem which the facade pattern solves is to simplify access to a set of interfaces in a subsystem. Facade solves this problem by providing a higher-level interface one level above all the sub-level interfaces. This higher-level interface then deals with the details and intricacies of the subsystem interface and at the same time provides a simplified interfaces to the clients of the subsystem.
Facade pattern improves decoupling between the clients and subsystems as the higher-level interface can absorb any changes which might happen in the lower subsystems interfaces and provide a consistent interface to the clients. This also has a direct benefit of system portability as the clients only design and code for the higher-level interface defined by the Facade. Porting of the subsystems then becomes easy as all the complexity of porting is hidden from the clients by the Facade layer.
Layered subsystems can have a facade for each layer. Thus communication between layers of the subsystem is much simplified as it happens through the subsystem facades rather than directly deal with the complexities of the subsystem's individual classes.
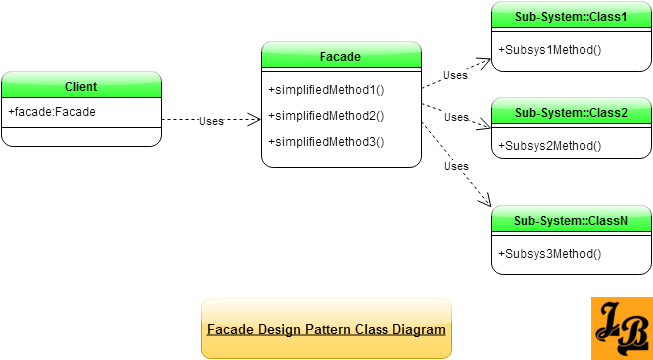
- The classes to the extreme right belong the Sub-System(depicted by the UML notation of ::).
- There are N subsystem classes-
Class1,Class2...ClassN
. - Facade layer, or
Facade
class as per this class diagram, invokes the sub-system classes as per the client request received by it on one of itssimplifiedMethods()
. SimplifiedMethods()
in turn invoke multiple subsystem classes based on the business logic.SimplifiedMethods()
may talk to multiple sub-system classes as per the business logic and in the end come up with the simplified/aggregated response which can be sent back to the client.- The
Client
class creates, or holds, an instance of theFacade
and invokes the specificsimplifiedMethod()
as per his needs. He just invokes the simplifiedMethod() and in return gets the simplified/aggregate response. Thus, theClient
is shielded from the underlying complexities of the sub-system layer by theFacade
.
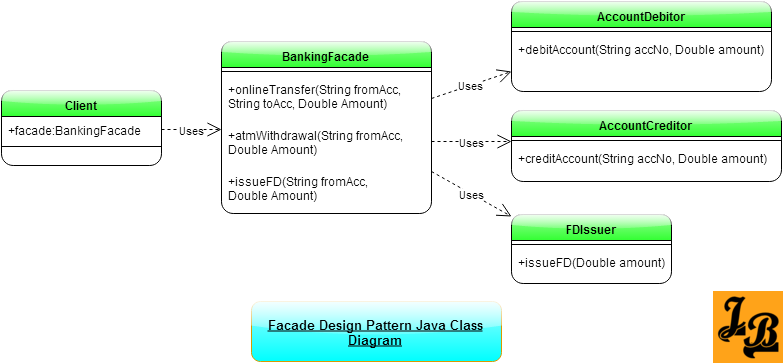
Code for the classes shown in Java Class Diagram
//AccountDebitor.java
public class AccountDebitor{
public void debitAccount(String accNo, Double amount){
//Logic for debiting 'amount' from account number 'accNo'
}
}
//AccountCreditor.java
public class AccountCreditor{
public void creditAccount(String accNo, Double amount){
//Logic for crediting 'amount' into account number 'accNo'
}
}
//FDIssuer.java
public class FDIssuer{
public void issueFD(Double amount){
//Logic for issuing FD for 'amount'
}
}
//BankingFacade.java
public class BankingFacade{
AccountCreditor creditor=new AccountCreditor();
AccountDebitor debitor=new AccountDebitor();
FDIssuer fdIssuer=new FDIssuer();
public void onlineTransfer(String fromAcc, String toAcc, Double amount){
debitor.debitAccount(fromAcc, amount);
creditor.creditAccount(toAcc, amount);
}
public void atmWithdrawal(String fromAcc, Double amount){
debitor.debitAccount(fromAcc, amount);
}
public void issueFD(String fromAcc, Double amount){
debitor.debitAccount(fromAcc, amount);
fdIssuer.issueFD(amount);
}
}
//Client.java
public class Client{
private BankingFacade facade;
public Client(BankingFacade facade){
this.facade=facade;
}
public static void main(String args[]){
BankingFacade facade=new BankingFacade();
facade.onlineTransfer("1001","1002",10000.00);
facade.atmWithdrawal("1001",1000.00);
facade.issueFD("1002",500.00);
}
}
AccountCreditor, AccountDebitor
andFDIssuer
are three classes in the banking sub-system which accomplish a functionality as represented by their name i.e. they credit an account with a specific amount, debit an account with the given amount, or issue an FD for a given amount respectively.BankingFacade
here holds handles to all the three sub-system classes. It invokes one or more of the sub-system classes based on the banking function requested by the Client.- For the operation online transfer on
BankingFacade
,Client
invokes just one methodonlineTransfer()
with debit-account, credit-account and amount to be transferred. InternallyBankingFacade
invokesAccountDebitor
to debit and thenAccountCreditor
to credit the amount. It also takes care of transactions and any exceptions which may happen.(I have not written code for transaction & exception handling in this example as it is not relevant to facade pattern as such). - Similar to onlineTransfer() method on BankingFacade, atmWithdrawal invokes a method of AccountDebitor. In the same way, issueFD() first invokes AccountDebitor to debit the amount of FD and then calls FDIssuer to issue the FD.
- The important thing to note is that the Client remains unaware of the complexities of
onlineTransfer(), atmWithdrawal()
orissueFD()
operations it invokes onBankingFacade
. This is because theBankingFacade
simplifies the underlying banking sub-system complexity by having simple input and output. But internallyBankingFacade
navigates through the sub-system complexity to make the banking operations work.
Gang of Four (GOF) Design Patterns on JavaBrahman
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern