Template Method Design Pattern in Java
This tutorial explains the Gang of Four Design Pattern named Template Method Pattern. It first defines the pattern, then via pattern's UML Class Diagram shows the various constituent classes in the pattern and explains the role of each of the classes. Next an example use case using Template Method Pattern is presented via its Class Diagram and Java code. Finally, the use case's class diagram and working of the Java code is explained in detail.
Introduction
Template Method Design Pattern is a behavioral design pattern among the Gang Of Four(GOF)Article on GOF Patterns & their types Design Patterns. I.e. the template method pattern deals with how the classes interact with each other.
What is Template Method Design Pattern
Template Method design pattern is used when the skeleton of an algorithm can be defined in terms of a series of operations, with few of these operations being redefined by sub-classes without changing the algorithm.
Lets say we have few steps to achieve something - say cooking an item. Few of the steps are common such as putting the gas stove on before cooking, putting the stove off after cooking etc. But some steps will be specific to that food item- for example some food will be boiled first then cooked, while some food will be roasted etc.
If we make a template(or, base class in programming terms) of an algorithm for cooking food, then we can implement the common methods defined earlier in the base class itself. At the same time we will leave the steps which are specific to the food item as abstract. These abstract methods will be implemented based on the recipe specific to the food item.
Now lets examine the basic structure of a template method pattern through its class diagram -
Template Method Design Pattern Class Diagram
Explanation of Class Diagram
Explanation of Code & Class Diagram
The Java Class Diagram is for a use case of an algorithm of cooking recipes for vegetables. Let us understand the Java code -
Lets say we have few steps to achieve something - say cooking an item. Few of the steps are common such as putting the gas stove on before cooking, putting the stove off after cooking etc. But some steps will be specific to that food item- for example some food will be boiled first then cooked, while some food will be roasted etc.
If we make a template(or, base class in programming terms) of an algorithm for cooking food, then we can implement the common methods defined earlier in the base class itself. At the same time we will leave the steps which are specific to the food item as abstract. These abstract methods will be implemented based on the recipe specific to the food item.
Template Method Design Pattern Class Diagram
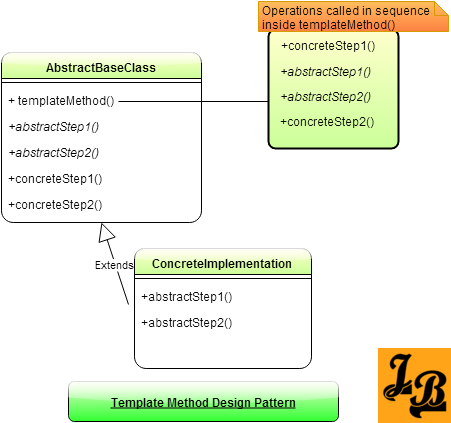
- This
AbtractBaseClass
class sews the whole algorithm together. - The
templateMethod()
is a concrete method which is responsible for calling all the 4 steps of the algorithm in correct order which isconcreteStep1()
,abstractStep1()
,abstractStep2()
andconcreteStep2()
. - Two of the steps in the algorithm are concrete named
concreteStep1()
andconcreteStep2()
. These concreteMethods have their implementation in the base class which is shared by all theConcreteImplementation
classes when they subclass theAbstractBaseClass
. - Two steps in the algorithm are abstract named
abstractStep1()
andabstractStep2()
. These abstract methods will be implemented by the specificConcreteImplementation
based on the needs of the concrete variant.
Note - You can have any number of concrete & abstract methods. I have taken 2 of each just for example.
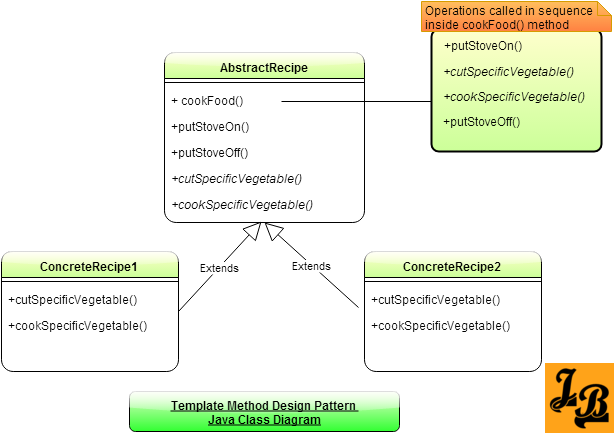
Code for the classes drawn in Java Class Diagram
//AbstractRecipe.java
public abstract class AbstractRecipe{
public void cookFood(){
putStoveOn();
cutSpecificVegetable();
cookSpecificVegetable();
putStoveOff();
}
public void putStoveOn(){
//code for putting stove on
}
public void putStoveOff(){
//code for putting stove off
}
public abstract void cutSpecificVegetable();
public abstract void cookSpecificVegetable();
}
//ConcreteRecipe1.java
public class ConcreteRecipe1 extends AbstractRecipe{
public void cutSpecificVegetable(){
//specific implementation for cutting as per recipe 1
}
public void cookSpecificVegetable(){
//specific implementation for cooking as per recipe 1
}
}
//ConcreteRecipe2.java
public class ConcreteRecipe2 extends AbstractRecipe{
public void cutSpecificVegetable(){
//specific implementation for cutting as per recipe 2
}
public void cookSpecificVegetable(){
//specific implementation for cooking as per recipe 2
}
}
-
AbstractRecipe
is the abstract base class in above use case. - 2 concrete methods in the
AbstractRecipe
areputStoveOn()
&putStoveOff()
. These contain implementation for putting the gas stove on and off. This implementation is independent of which vegetable is being cooked and hence is implemented in the base class and is inherited as-is by the child classes. - The
templateMethod()
iscookFood()
which calls all the 4 methodsputStoveOn()
,cutSpecificVegetable()
,cookSpecificVegetable()
andputStoveOff()
in order. - There are 2 concrete implementation classes called
ConcreteRecipe1
andConcreteRecipe2
- The concrete implementation classes implement their own versions of
cutSpecificVegetable()
&cookSpecificVegetable()
based on the specific recipe needs.
Gang of Four (GOF) Design Patterns on JavaBrahman
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern