Java 8 - Iterable.forEach, Iterator.remove methods tutorial with examples
Collections API is the most popular and widely used utility API in the Java universe. With the advent of Functional Interfaces, Lambdas and Streams in Java 8, Collections API has also undergone changes to accomodate and build-upon the newly introduced functional programming features. The most widely used Collection classes and interfaces such as Iterable and Iterator, Collection, List and Map have all been enhanced in JDK 1.8 with new features and methods.
This is the 1st article in a 4 part article series in which I will cover the changes introduced in Java 8 Collections in detail.
In this part 1 of 4, I will be covering the new default method named
Java 8's new
Where,
-
-
Let us now take a look at Java code showing how to use
OUTPUT of the above code
Explanation of the code
New default method
As a result, the practice of overriding the
The default implementation of
As you can see in the above code, the default implementation of
In the forthcoming parts I will be taking you to the depths of Java 8 Collections enhancements, where I will explain the changes in important Collection classes viz.
This is the 1st article in a 4 part article series in which I will cover the changes introduced in Java 8 Collections in detail.
In this part 1 of 4, I will be covering the new default method named
forEach()
introduced in java.lang.Iterable
interface with examples. This will be followed by understanding the new default implementation of Iterator interface's remove()
method, and how it makes implementing the Iterator easier than before.
New default method forEach()
added to the Iterable Interface in Java 8
Iterable
interface is a commonly extended interface among the Collections interfaces as it provides the ability to iterate over the members of a collection. Collection
, List
and Set
are among the important Collections interfaces that extend Iterable
, apart from other interfaces.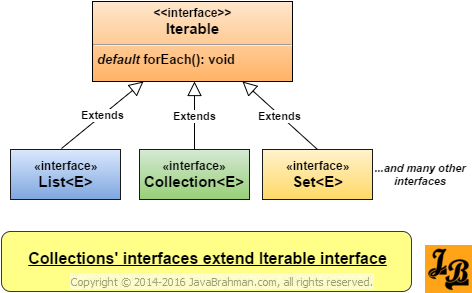
Iterable.forEach()
method has the following signature - default void forEach(Consumer<? super T> action)
-
action
is the only parameter and is an instance of a Consumer Functional InterfaceClick to read detailed tutorial on Consumer Functional Interfaces-
forEach()
is implemented in the Iterable
interface itself as a default methodTutorial explaining the concepts of Java 8's new default methods.Iterable.forEach()
method 'consumes' all the elements of the iterable collection of elements passed to it. The logic for consumption is passed to the method as an instance of a Consumer functional interface. An important point to note is that the forEach method iterates internally over the collection of elements passed to it rather than externally. You can read about declarative internal iterators and how they differ from commonly used external iterators in this tutorial hereClick to read detailed tutorial explaining internal vs external iterators.Let us now take a look at Java code showing how to use
Iterable.forEach()
method for consuming the elements of an Iterable
collection -
Java 8 code to iterate and consume using Iterable.forEach() method
package com.javabrahman.java8.collections;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class IterableForEachExample {
public static void main(String args[]){
List<Integer> intList= Arrays.asList(12,25,9);
System.out.println("List elements printed using Iterable.forEach");
intList.forEach(System.out::println);
Set<Integer> intSet=new HashSet<>();
intSet.add(50);
intSet.add(1);
System.out.println("Set elements printed using Iterable.forEach");
intSet.forEach(System.out::println);
}
}
List elements printed using Iterable.forEach 12 25 9 Set elements printed using Iterable.forEach 1 50
- A
List
of primitiveint
s, namedintList
, is created using theArrays.asList()
method. forEach()
method is invoked on theList
instance -intList
. As we read above,List
inherits theforEach()
method's default implementation fromIterable
interface.- We pass a method referenceClick to read tutorial on Java 8 Method References to
System.out.println()
method, which is aConsumer
type of function, as parameter to theforEach()
method. forEach()
method internally iterates and consumes, or prints, the elements ofintList
.- Next we created an instance of a
Set
, namedintSet
, by using its concrete implementationHashSet
. Set
also inheritsIterable
. Hence, we are able to print the elements inintSet
using theforEach()
method similar to the way we did withintList
.
remove()
added to Iterator
interface in Java 8
Prior to Java 8, implementing Iterator
interface without the support for remove()
method implied that the designers had to override the method and throw an UnsupportedOperationException
. Such an override was commonly done and over the years had become kind of staple in Iterator
implementations not supporting the remove operation.
With Java 8 arrived the feature of adding default implementations of methods in interfaces itself. Java designers have used this new feature and added a default implementation of the remove()
method in the Iterator
interface itself which throws UnsupportedOperationException
.As a result, the practice of overriding the
remove()
method, whenever it wasn't supported, has now been inverted to overriding the remove()
method only when remove functionality has to be implemented. This has removed the unnecessary overhead of overriding and throwing the UnsuportedOperationException
everytime when implementing an Iterator
without remove functionality.The default implementation of
remove()
method in Java 8's Iterable
is as shown below - default void remove() {
throw new UnsupportedOperationException("remove");
}
Iterator.remove()
method just throws an UnsupportedOperationException
with message "remove"
.
Conclusion
In this tutorial we dipped our feet into the waters of Java 8 Collection Enhancements by taking a look at the changes in Iterable
and Iterator
interfaces.In the forthcoming parts I will be taking you to the depths of Java 8 Collections enhancements, where I will explain the changes in important Collection classes viz.
Collection
, List
and Map
interfaces. Specifically, among the Map
enhancements we will take a look at the new methods added in Java 8 which make multi-value maps handling easier than before.Continue Reading on Java 8 Collection Enhancements
Part 1 - Iterable.forEach, Iterator.remove methods tutorial with examplesRead Java 8 Collection Enhancements- Part 1Part 2 - Collection.removeIf method tutorial with examplesRead Java 8 Collection Enhancements- Part 2Part 3 - List.sort, List.replaceAll methods tutorial with examplesRead Java 8 Collection Enhancements- Part 3 Part 4 - Map's computeIfAbsent, computeIfPresent, getOrDefault methods tutorial with examplesRead Java 8 Collection Enhancements- Part 4
Part 1 - Iterable.forEach, Iterator.remove methods tutorial with examplesRead Java 8 Collection Enhancements- Part 1Part 2 - Collection.removeIf method tutorial with examplesRead Java 8 Collection Enhancements- Part 2Part 3 - List.sort, List.replaceAll methods tutorial with examplesRead Java 8 Collection Enhancements- Part 3 Part 4 - Map's computeIfAbsent, computeIfPresent, getOrDefault methods tutorial with examplesRead Java 8 Collection Enhancements- Part 4