Understanding Thread Life Cycle, Thread States in Java | Tutorial with Examples
This tutorial explains thread life cycle in Java with examples. This is the second article in the Java Concurrency Series, with the first articleClick to Read tutorial on Java MultiThreading Basics & How-to create/run Threads covering basics of multithreading in Java. In this tutorial we will start by looking at the Java thread lifecycle diagram. We will then look at individual thread states in detail to understand the state information they encapsulate and how transitions happen between these states. Lastly, we will take a look at a code example showing how a thread moves through its states, understand the logic of the program via a sequence diagram and then understand how the code works.
Java Thread Life Cycle
Let us start by getting a high level understanding of the 6 thread states in Java with the diagram shown next -
Above diagram shows how a typical thread moves through the different stages of its life cycle. Let us look at these states one-by-one and understand their place in the life of a Java thread -
In-built Java Enum Constants for thread states
To provide a standard naming and reference for individual thread states, Java language designers have defined an enum named
OUTPUT of the above code
Explanation of the code
To better understand how
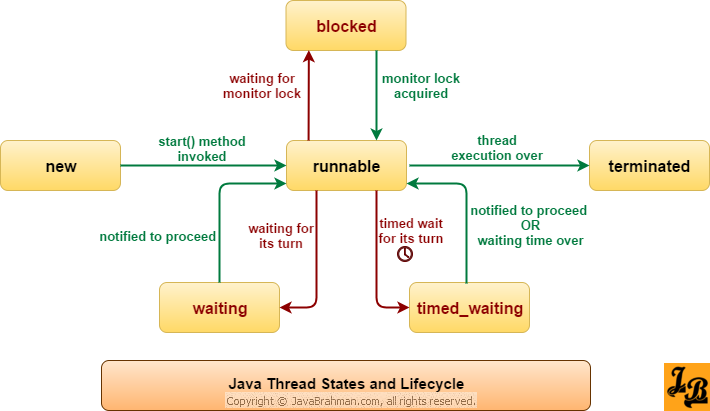
- New - A newly created thread object instance on which the
start()
method has not yet been invoked is in thenew
state. To learn how to instantiate threads in the proper way check out this tutorialClick to Read tutorial explaining how to create a Thread in Java. - Runnable - A thread in
new
state enters therunnable
state when theThread.start()
method is invoked on it. There are 2 important points to note regarding therunnable
state -- Although the thread enters the
runnable
state immediately on invoking thestart()
method, but it is not necessary that the thread immediately starts executing. A thread runs when the logic it holds in itsrun()
method can be executed by the processor. In case the thread logic needs any resource which is not available then the thread waits for the resource to become available. Secondly, a thread in
runnable
state may run for some time and then get blocked for a monitor lock, or enter thewaiting
/timed_waiting
states as it waits for the opportunity/time to enterrunnable
state again.
- Although the thread enters the
- Blocked - A running thread may enter the
blocked
state as it waits for a monitor lock to be freed. It may also beblocked
as it waits to reenter a monitor lock after being asked to wait using theThread.wait()
method. - Waiting - A thread enters the
waiting
state when it is made to wait for a go-ahead signal to proceed. The go-ahead in this case is given by another thread and can be given in the following 3 scenarios -- Thread waiting due to
Thread.wait()
method being called on it: The other thread can useThread.notify()
orThread.notifyAll()
to give the go-ahead to thewaiting
thread. - Thread waiting as it itself has asked for joining another thread using
Thread.join()
: Thewaiting
thread gets a go-ahead when the thread its waiting for ends. Thread waiting due to
The waiting thread resumes whenLockSupport.
park()
method being invoked on it:LockSupport.
unPark()
is called with the parked thread object as the parameter.
- Thread waiting due to
- Timed_Waiting - A thread which is waiting as it has been specifically 'instructed' to wait for a specified waiting time is in a
timed_waiting
state. A thread can be made to wait for a pre-determined amount of time in the following ways -- Thread made to wait using
Thread.sleep()
method. - Threads being asked to wait for a permit for a specified amount of time using
LockSuport.parkNanos()
andLockSupport.parkUntil()
methods. Threads being made to wait for a fixed amount of time using
Thread.wait(long millis)
orThread.join
(long millis, int nanos)
.
- Thread made to wait using
- Terminated - A thread enters its 'final resting' state or
terminated
state when it has finished executing the logic specified in itsrun()
method.
java.lang.Thread.State
which has the following constants defined (each one named after the thread state it refers to) -
ThreadState.BLOCKED
ThreadState.NEW
ThreadState.RUNNABLE
ThreadState.TERMINATED
ThreadState.TIMED_WAITING
ThreadState.WAITING
Java example showing how a thread moves between different states
package com.javabrahman.corejava.threads;
public class ThreadStates {
public static void main(String args[]){
//Creating an instance of Basic Thread
Thread threadInstance=new Thread(new BasicThread());
threadInstance.start();
System.out.println("BasicThread State: "+threadInstance.getState());
try {
boolean keepRunning=true;
int count=1;
while(keepRunning) {
Thread.sleep(2000);
System.out.println(count*2+ " Seconds elapsed - BasicThread State: "+threadInstance.getState());
count++;
if(count==4){
//6 seconds elapsed
synchronized(threadInstance) {
threadInstance.notify();
}
}
if(Thread.State.TERMINATED == threadInstance.getState()){
keepRunning = false;
}
}
}catch(InterruptedException iException){
iException.printStackTrace();
}
}
}
//BasicThread.java
package com.javabrahman.corejava.threads;
public class BasicThread implements Runnable {
@Override
public void run() {
Thread thread = Thread.currentThread();
try{
//Making the thread sleep for 5 seconds
System.out.println("Basic thread to sleep for 5 seconds");
thread.sleep(5000);
synchronized (thread) {
thread.wait();
}
}catch(InterruptedException iException){
iException.printStackTrace();
}
}
}
BasicThread State: RUNNABLE Basic thread to sleep for 5 seconds 2 Seconds elapsed - BasicThread State: TIMED_WAITING 4 Seconds elapsed - BasicThread State: TIMED_WAITING 6 Seconds elapsed - BasicThread State: WAITING 8 Seconds elapsed - BasicThread State: TERMINATED
BasicThread.java
is moving through different thread states, let us first take a look at a sequence diagram showing the interactions between above two programs - 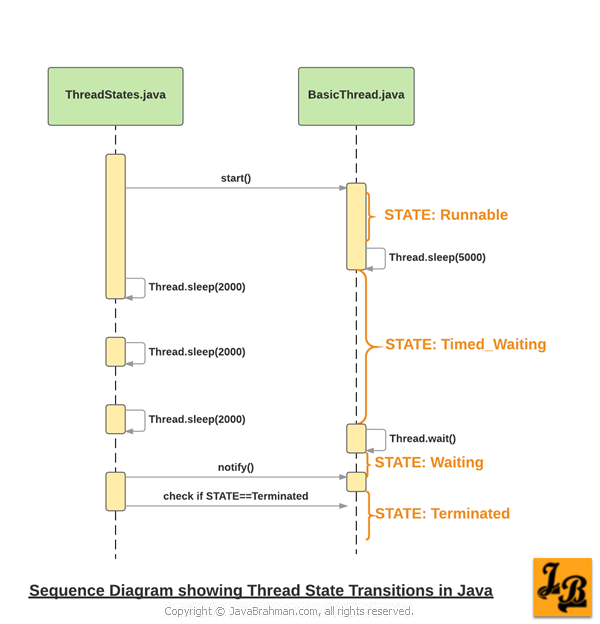
ThreadStates.java
is the class with themain()
method in above example. It instantiates aBasicThread.java
instance, namedthreadInstance
,and then callsstart()
onthreadInstance
to start the execution of parallel thread. The state ofBasicThread
is printed at this point asRUNNABLE
.ThreadStates
then gets into an infinite while loop with a boolean flag variable namedkeepRunning
. At the beginning of the loop the thread goes to sleep for2000
milliseconds
or 2 seconds. At the same timeBasicThread
too goes to sleep for5000
milliseconds
.- In every iteration of while loop, at a gap of every 2 seconds,
BasicThread
’s state is printed. During 1st iteration(2 seconds) and 2nd iteration(4 seconds) elapsed times -BasicThread
is printed as being in stateTIMED_WAITING
as it is sleeping for 5 seconds. - When 5 seconds elapse
BasicThread
wakes up, and it immediately asks itself to wait usingThread.wait()
method. The state ofBasicThread
is printed at this point asWAITING
. - Then, in 3rd iteration i.e. when 6 seconds elapse,
ThreadStates
invokesnotify()
onBasicThread
which then starts executing. - In 4th iteration(8 seconds)
ThreadStates
checks forBasicThread
’s state. The state is nowTERMINATED
. The flagkeepRunning
is now set to false.ThreadStates
exits the while loop and ends its processing.