Java 8 Multiple Inheritance of Behavior from Interfaces using Default Methods
This article explains how default methods enable multiple inheritance of behavior in Java 8 using the new default methods feature in Interfaces.
Multiple Inheritance of Behavior with Default Methods
Until Java 7 multiple interface inheritance was possible but interfaces were not allowed to have concrete methods. Thus, behavior inheritance was not possible. With Java 8, where interfaces can now have default methods
Click to Read Default Methods tutorial implemented in them, it is now possible to have a derived class inherit methods from multiple parent interfaces. So, multiple inheritance of behavior is now possible.
Example to understand multiple inheritance using default methods
In the above class diagram -
OUTPUT of the above code
Explanation of the code
I have covered conflict resolution rules for such scenarios and the classic diamond problem resolution in my next article which you can read hereRead tutorial on Java 8 multiple inheritance conflict resolution and diamond problem.
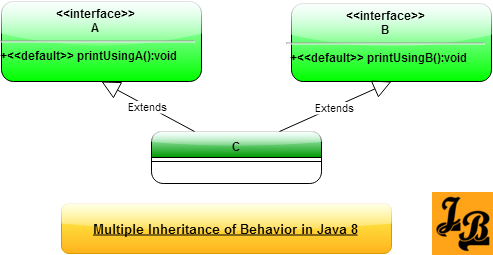
- A is an interface with a default implemented method
printUsingA()
. - B is an interface with a default implemented method
printUsingB()
. - C is a concrete class implementing both A and B interfaces.
Java 8 code showing multiple inheritance for interfaces A,B & class C
//A.java
public interface A {
public default void printUsingA() {
System.out.println("Print from A");
}
}
//B.java
public interface B {
public default void printUsingB() {
System.out.println("Print from B");
}
}
//C.java
public class C implements A,B {
public static void main(String args[]) {
C cObj=new C();
cObj.printUsingA();
cObj.printUsingB();
}
}
Print from A Print from B
- In the
main()
method of C an instance of C called cObj is created. - Methods
printUsingA()
&printUsingB()
are invoked on cObj. - Multiple inheritance of behavior is thus achieved as C inherits 2 implemented methods(or behaviors from A & B).
I have covered conflict resolution rules for such scenarios and the classic diamond problem resolution in my next article which you can read hereRead tutorial on Java 8 multiple inheritance conflict resolution and diamond problem.